Table of Contents
Python tkinter
In these days, Python is a very popular programming language to develop various kinds of applications, AI systems, games etc. In Python, Python tkinter is a powerful module to create Graphical User Interface (GUI) for almost all kinds of platforms like Windows, Unix/Linux and MAC.
For the present time people like to work with GUI instead of CLI. So, GUI is the most popular interface among all kinds of programmers. GUI gives a graphical view to the users of the application to deal with it in a very simple way. You can read the full documentation of python tkinter from here.
User Registration Form in Python tkinter
The applications that have security concern need to introduce Login systems to enter into the main interface of the application. In this system, users need to enter their username and password to login into the system.
For this, users need to register themselves into the system to create their user accounts. After creating their user accounts, they will be able to login into the system. You can learn to create a login page in python tkinter by reading how-to-create-login-form-in-python-tkinter.
For this purpose, a developer needs to add a Registration Form for his/her users to register into the system. In the registration form, a user should enter some personal information like their Full name, email address, phone number, password etc.
After entering all the required data, they should click on the Register button. After clicking on that button, the user information will be saved to the database of the server. It means, the registration is completed.
After the successful registration, users can log into the application with their proper credentials after the verification of the details entered by them. Some details should be verified by the admin like email, phone number etc. In some cases, captcha verification is also needed.
User Registration Form in Python tkinter looks like this
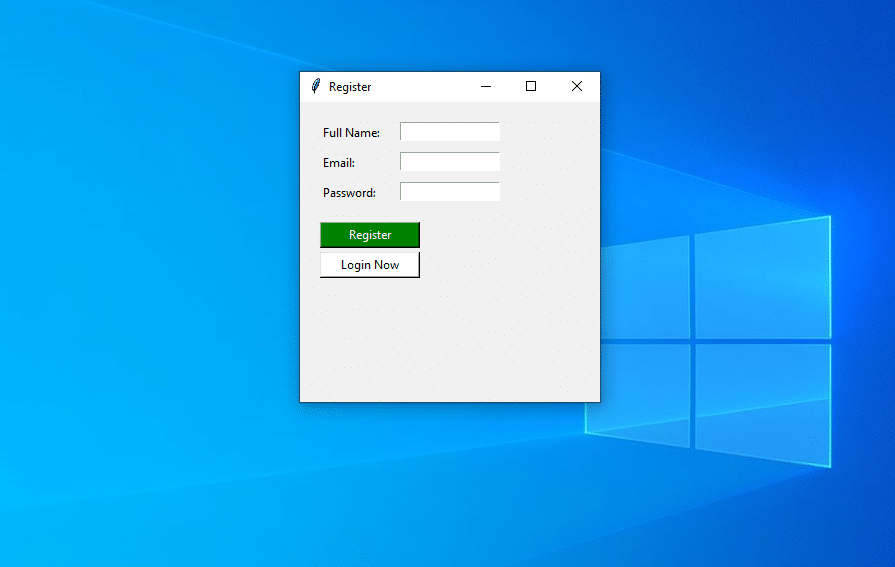
In the above python tkinter User Registration Form example, three fields are added namely Full name, email address and password. After that two buttons have been added. They are Registration button and login button.
A user can click on registration button after entering all the required details in the given entry boxes. The registration process will begin after clicking on that button.
Login button is given for the users to get logged in if they are already registered under the application. By clicking this button, they will be taken to the login form. From where they will be able log in into the system after entering correct user credentials.
Code to create User Registration Form in Python tkinter
import tkinter as tk
from tkinter import *
r = tk.Tk()
r.geometry("300x300")
r.title("Register")
lbl1 = tk.Label(r, text="Full Name: ")
lbl1.place(x = 20, y = 20)
fullname = tk.Entry(r, width="35")
fullname.place(x = 100, y = 20, width = 100)
lbl2 = tk.Label(r, text="Email: ")
lbl2.place(x = 20, y = 50)
email = tk.Entry(r, width="35")
email.place(x = 100, y = 50, width = 100)
lbl3 = tk.Label(r, text="Password: ")
lbl3.place(x = 20, y = 80)
password = tk.Entry(r, show="*", width="35")
password.place(x = 100, y = 80, width = 100)
registerBtn = tk.Button(r, text="Register", bg="green", fg="#ffffff")
registerBtn.place(x = 20, y = 120, width = 100)
loginBtn = tk.Button(r, text="Login Now", bg="#ffffff", fg="#000000")
loginBtn.place(x = 20, y = 150, width = 100)
r.mainloop()
Above code will help you to create a simple user registration using Python tkinter. You can make required alteration if you need. Color codes and length/width etc. can be changed. In the above code, backend part is not included. In the future posts, the backend parts of the registration and login will also be given. Please stay connected with us.
In the above code, only design part is discussed. Designing is the first step of creating a registration form. Try to learn this design code and implement it. You can make required changes to create a more attractive design for your user registration form.
Thank you…
Happy Coding…